Best 5 Effective Ways to Reverse a List in Python
Reversing a list is a common task in programming, particularly in Python. As a dynamic and versatile language, Python offers multiple methods to reverse a list, making it a useful skill for any programmer, especially beginners. This article outlines five effective ways to reverse a list in Python, exploring different techniques and their applications. Understanding how to manipulate lists, including the reversal of items, is essential for mastering Python data structures.
By learning these various methods, you can enhance your coding skills and improve your Python programming practices. Each method has its unique advantages, making some more suitable than others depending on your specific programming needs. As we delve into list reversal techniques, you will see practical examples that illustrate these concepts in action. Read on to uncover the best practices for reversing lists in Python while reinforcing your understanding of Python programming basics.
Using the Built-in Reverse Function
The first method to reverse a list in Python is by using the built-in reverse()
function. This function reverses the elements of the list in place, modifying the original list. It's simple and efficient for cases where you don't need to keep the original order of the list.
Here’s a brief example:
my_list = [1, 2, 3, 4, 5]
my_list.reverse()
print(my_list) # Output: [5, 4, 3, 2, 1]
When using this method, it’s good to remember that the operation affects the original list. If you are looking to keep the original list intact while reversing it, you may want to consider other methods. This basic technique is essential when learning Python list functions and how lists can be manipulated.
Common Mistakes with the Reverse Function
A common mistake when using the reverse()
function is attempting to assign its output to another variable, which can lead to confusion:
reversed_list = my_list.reverse()
This will result in reversed_list
being None
because reverse()
doesn't return a new list. Instead, it returns None
after altering the original list. Always check the return value of list methods in Python.
Utilizing List Slicing for Reversal
Another effective technique for reversing a list is using list slicing. Slicing creates a new list and allows you to specify a negative step to reverse the elements. This method is elegant and concise, making it an ideal choice when working with Python list applications.
Here’s how slicing can reverse a list:
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1]
print(reversed_list) # Output: [5, 4, 3, 2, 1]
This slicing technique is beneficial as it maintains the original list’s order while creating a new reversed version. It's also a great application of Python list handling capabilities.
Performance Considerations
While slicing creates a new list, it’s critical to consider the performance implications, especially with larger datasets. Creating copies involves additional memory overhead, which can lead to decreased performance when working with extensive collections. For larger lists or performance-critical applications, using the reverse()
function would be preferable.
Employing the Reversed() Function
Python's built-in reversed()
function is another approach to reverse a list. Unlike reverse()
, it returns an iterator, thus preserving the original list while still providing the reversed order. This is a particularly useful feature when you want to iterate over the list in reverse.
An example of using reversed()
is as follows:
my_list = [1, 2, 3, 4, 5]
reversed_iterator = reversed(my_list)
print(list(reversed_iterator)) # Output: [5, 4, 3, 2, 1]
This approach not only avoids modifying the original list but also illustrates a common pattern in Python programming with regard to manipulating collections. It’s a great way to integrate reversed order in Python operations.
Iterating with Reversed()
Using reversed()
allows for efficient looping without the need to create a new list entirely:
for item in reversed(my_list):
print(item)
This technique is particularly beneficial in sorting lists in Python where you might want to process items in reverse order without altering the existing data structure.
List Comprehension for Reversal
For those who love compact code, list comprehension offers a unique way to reverse a list. By leveraging this feature in Python, you can create a new reversed list in a single line:
my_list = [1, 2, 3, 4, 5]
reversed_list = [my_list[i] for i in range(len(my_list) - 1, -1, -1)]
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Although it’s not the most efficient way to reverse a list, especially with larger datasets, it displays the versatility of Python list manipulation in a more idiomatic manner. This method is also a great illustration of programming in Python.
Best Practices for List Comprehension
While using list comprehension, ensure that you are aware of its performance implications. It is essential for Python for beginners to understand that concise code does not always correlate with efficiency. Before fully adopting list comprehension for reversing lists in Python, consider the context and the importance of performance optimization in data handling patterns.
Using the Sorted Function for Custom Reversal
Beyond traditional reversal, the sorted()
function allows for creative list manipulation, including reversal through custom key functions. By harnessing the power of sorting techniques, you can reverse the order based on specific criteria.
my_list = [5, 3, 1, 4, 2]
sorted_list = sorted(my_list, reverse=True)
print(sorted_list) # Output: [5, 4, 3, 2, 1]
This method involves more than just reversing; it highlights the functionality of sorting lists in Python while simultaneously achieving the result of a reversed order when needed. Such flexibility is essential for data manipulation in Python.
Comparative Observations
It’s essential to consider that while the sorted()
function is handy, it does not perform a true reversal of the list's current order but rather sorts it based on the specified conditions. Understanding the differences between these methods can significantly improve your Python coding standards and practices.
Conclusion
Knowing how to reverse a list in Python enhances your list manipulation capabilities and lays a foundation for more advanced programming techniques. The built-in reverse()
function, list slicing, reversed()
, list comprehension, and the sorted()
function all provide diverse methods to achieve this crucial task.
As you develop your coding skills, consider which method aligns best with your programming needs—whether it’s efficiency, simplicity, or preserving the original data structure. Mastery of these techniques can enhance your Python projects, making data handling more efficient and effective. To further your Python journey, explore additional resources and tutorials to continue building your skills.
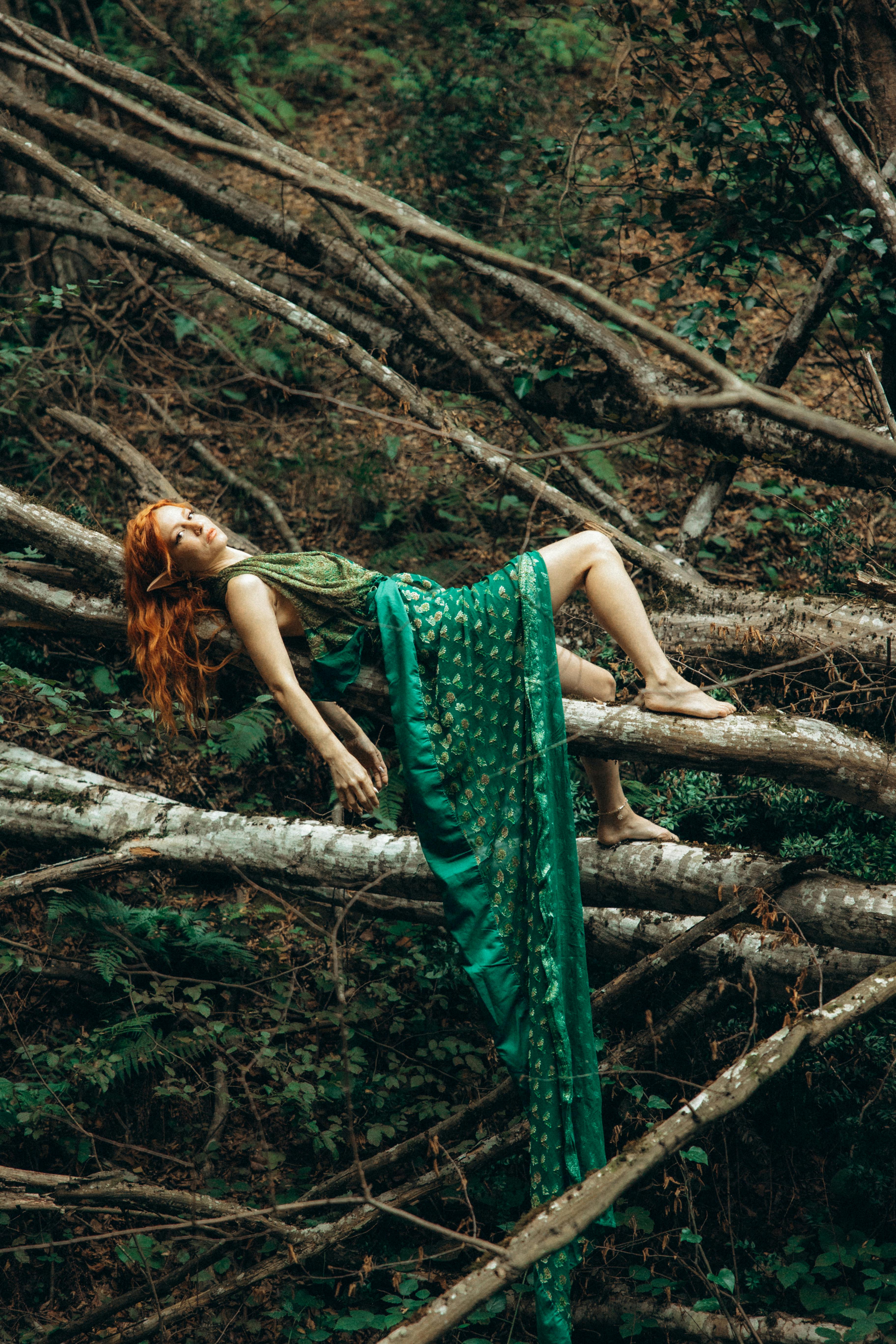
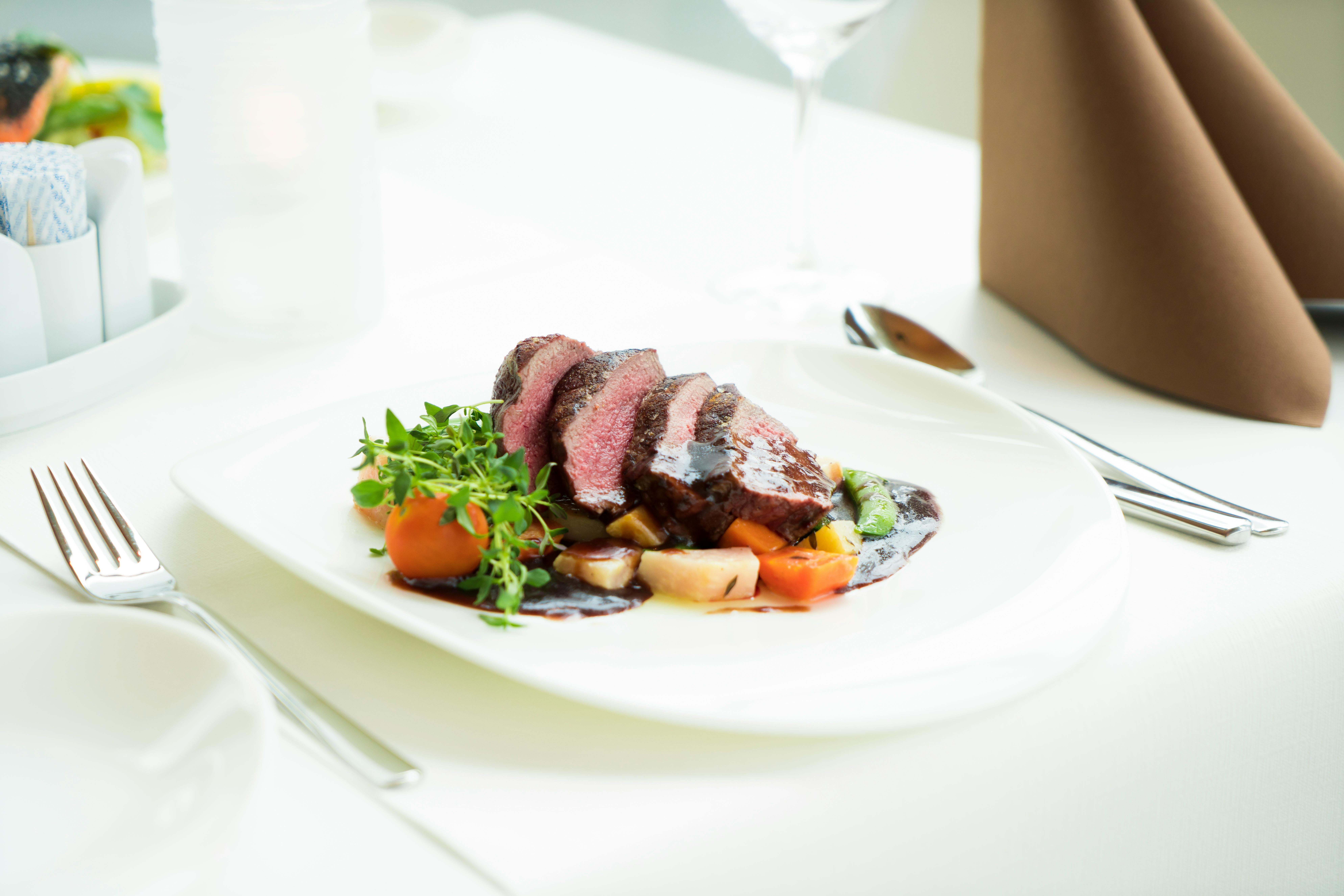